It might be easier to maintain this project as you originally outlined it if you maintained all your Search/Replace commands in a single text document. Then you could have Keyboard Mastro to access this text document and apply it's commands to whatever document you wish abbreviate.
Your current approach requires you to have an ever expanding Keyboard Maestro macro. Each Search/Replace that you wish to use requires yet another line in the macro which will soon be unwieldily as it continually grows.
An alternative is to maintain a text document. Each line in that document specifies a Search/Replace command. The macro goes through this document, line by line, and applies the commands to the text that you are trying to abbreviate. You can "test" each pattern before you add it. Once you are confident that it is appropriate, you can add it to the document as a new line. And it makes sense to use Regex.
This Search/Replace document can be formatted as
Search Text TAB Replace Text
Search Text TAB Replace Text
Search Text TAB Replace Text
etc.
The Keyboard Maestro macro goes through this text, line by line, and extracts the Search Text and extracts the Replace Text and applies them to the document that you are trying to abbreviate.
The Search Text should be a Regex (regular expression) pattern which will make your goals easier. If you are simply trying to replace the sequence of characters dog with the sequence of characters cat you can do that, but more commonly you will be wanting the replace the word dog with the word cat and a more complicated Regex pattern will be appropriate. With something simple like associated with TAB a/w, Regex works just like a simple search and replace.
SAMPLE SEARCH/REPLACE DOCUMENT
\band\b &
\bbilateral\b B/L
associated with a/w
\bthis\b
\bis\b
Using a text editor like BBEdit, allows you to "see" invisible characters like TAB (the red triangle) and SPACE (the red dot). Otherwise it is hard to know exactly what your document contains. The last line contains only spaces and TAB and is not visible when looking at the plain text but it is there. (See image below)
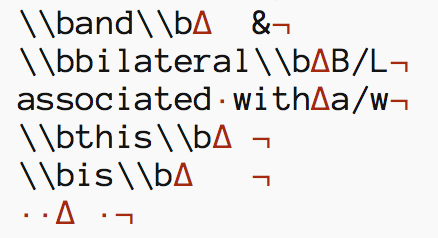
Things to note.
-
The Regex command to search for the word "and" is \band\b. \b is the Regex command to find the beginning or end of a word. I could not get this to work in my script without escaping the \ . I am not sure why this is. (Anyone?). However if you escape the \ then it will work. To escape it, you have to double the \ That is why instead of
\band\b
the document contains
\\band\\b
-
The last line replaces double spaces with single spaces. Double spaces start accumulating when you replace some word like "is" with nothing.The spaces that were originally on both sides of "is" now are joined as a double space. This last line cleans this up. It is possible that you might have to do this more than once to get all the double spaces. I did not test this
HOW DOES THE MACRO WORK?
- The first line just assigns the contents of the Search/Replace document to the variable MySrchRplc. If you update your Search/Replace document, you would update this first line.You cannot see the TAB characters, but they are there.
Set Variable “MySrchRplc” to Text
\\band\\b &
\\bbilateral\\b B/L
associated with a/w
\\bthis\\b
\\bis\\b
- Before running the macro, you put the document that you wish to shorten onto the Clipboard. The second line takes that document you are trying to abbreviate and assigns it to the variable TextToShorten.
Set Variable “TextToShorten” to Text
%SystemClipboard%
- The third line initiates a for loop going through each line of the Search/Replace document.
For Each Item in the Collection Execute Actions
The lines in Variable “MySrchRplc”
Execute the Following Actions:
- & 5.The fourth and fifth lines use some Regex magic to extract the appropriate text into the SearchFor and ReplaceWith variables. For the first line in the search/replace document SearchFor is assigned \band\b and ReplaceWith is assigned &.
Search Variable “SingleLine” Using Regular Expression (ignoring case)
Search for “(^[^\t]*)”
And capture to:
Ignored
SearchFor
Stop macro and notify on failure.
Search Variable “SingleLine” Using Regular Expression (ignoring case)
Search for “([^\t]*$)”
And capture to:
Ignored
ReplaceWith
Stop macro and notify on failure.
- The sixth line does the actual work of going through the TextToShorten document and making the appropriate substitutions.
Search and Replace Variable “TextToShorten” Using Regular Expression (ignoring case)
Search for “%Variable%SearchFor%”
Replace with “%Variable%ReplaceWith%”
Notify on failure.
- The seventh line displays the now abbreviated document.
Display Text in Window
%Variable%TextToShorten%
StripUselessV2.kmmacros (4.4 KB)