@skillet, here is my Macro that uses the JavaScript querySelectorAll
, rather than Xpath, to extract the links from the Google Search page.
I have found that usually it is much easier to use querySelectorAll
than Xpath.
That is still so in this case, but Google has made it much harder with their latest changes.
My macro seems to work well (tested with Google Chrome 88.0.4324.96 (4324.96) on macOS 10.14.6 (Mojave)) and should work fine in Safari as well.
Be aware that ==this script could fail if Google changes key elements on this web page.==
Note that the results of the Chrome Search page can vary in format/layout based on what you search for. I have excluded links in the subsection of the first result, and all links in the "People Also Ask" section:
The JavaScript in my Macro returns ALL of the UNIQUE primary links found on the Search Results page.
This allows you to easily control in the Macro itself how many links you want to process.
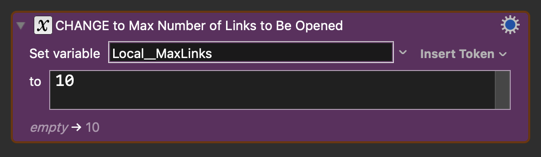
I use the KM For Each action to open each URLs until the max number is reached. This uses the KM Open URL set to use the "Default App" (which will be your default Web Browser), but you can easily change it to open in a specific Browser, and/or to open in a new window or new tab -- whatever you prefer:
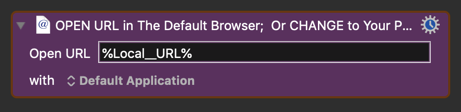
I also provided a disabled Action to display the list of links, in case you want to just examine the list or debug.
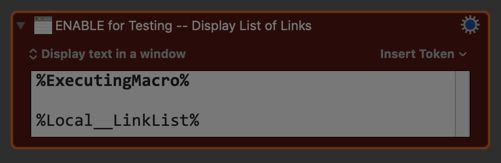
Please let me know if you have any problems with this macro.
Below is just an example written in response to your request. You will need to use as an example and/or change to meet your workflow automation needs.
Please let us know if it meets your needs.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
MACRO: Extract Unique Links from Google Search [Example]
-~~~ VER: 1.0 2021-01-27 ~~~
Requires: KM 8.2.4+ macOS 10.11 (El Capitan)+
(Macro was written & tested using KM 9.0+ on macOS 10.14.5 (Mojave))
DOWNLOAD Macro File:
Extract Unique Links from Google Search [Example].kmmacros
Note: This Macro was uploaded in a DISABLED state. You must enable before it can be triggered.
ReleaseNotes
Author.@JMichaelTX
PURPOSE:
- Extract Unique Links from Google Search [Example]
- Works with Safari, Google Chrome, and any Chrome-based Browser
- Extracts Links from the Front-Most Browser
HOW TO USE
- First, make sure you have followed instructions in the Macro Setup below.
- Open Web Browser with Google Search Results
- Trigger this macro.
- This Macro Could Fail If Google Makes Key Changes to its Search Page
- Developed using Google Chrome 88.0.4324.96 (4324.96) on macOS 10.14.6 (Mojave)
MACRO SETUP
- Carefully review the Release Notes and the Macro Actions
- Make sure you understand what the Macro will do.
- You are responsible for running the Macro, not me. ??
.
Make These Changes to this Macro
- Assign a Trigger to this macro.
- Move this macro to a Macro Group that is only Active when you need this Macro.
- ENABLE this Macro, and the Macro Group it is in.
.
- REVIEW/CHANGE THE FOLLOWING MACRO ACTIONS:
(all shown in the magenta color)
- CHANGE to Max Number of Links to Be Opened
- OPEN URL in The Default Browser; Or CHANGE to Your Preferred Browser
REQUIRES:
- KM 9.0+ (may work in KM 8.2+ in some cases)
- macOS 10.11.6 (El Capitan)+
TAGS: @JSIB @GoogleChrome @URL @Links @Example
USER SETTINGS:
- Any Action in magenta color is designed to be changed by end-user