No, that's not how Try/Finally works.
Try/Finally with Error in the Try Section
The "After try/finally"
console message does not get displayed.
try {
console.log("Starting");
throw new Error("Example error");
} finally {
console.log("Finally");
}
console.log("After try/finally");
Result:
/* Starting */
/* Finally */
Result:
Error -2700: Script error.
NOTE: If you run the script in Script Editor, don't forget to have it show the "Replies":
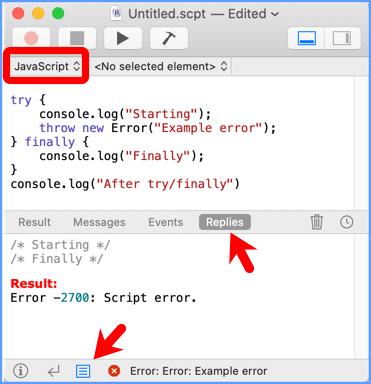
So, for our Prompt code, this guarantees that the prompt gets closed no matter that else happens, but it still aborts if there's an error:
try {
showPrompt();
throw new Error("Example error");
} finally {
closePrompt();
}
console.log("After try/finally");
Here's some other examples, which will answer some questions I'm guessing you might have:
Baseline Case with No Errors
Nothing surprising here, but I included this to be complete.
try {
console.log("Starting");
} finally {
console.log("Finally");
}
console.log("After try/finally");
Result:
Starting
Finally
After try/finally
Try/Finally with Return
In KM, the "return" could also be something like "Cancel Macro". Again, the "After try/finally"
console message does not get displayed.
tryFinallyWithReturn();
function tryFinallyWithReturn() {
try {
console.log("Starting");
return "Exiting";
} finally {
console.log("Finally");
}
console.log("After try/finally");
}
Result:
/* Starting */
/* Finally */
Result:
"Exiting"
Try/Finally with Error, and Error in Finally
The error message from the error in the Try section gets eaten, replaced with the error message from the error in the Finally section
try {
console.log("Starting");
throw new Error("Example error");
} finally {
console.log("Finally");
throw new Error("Finally error");
}
console.log("After try/finally");
NOTE: You can't see the results clearly in Script Editor, so I'm showing the results from VSCode, slightly edited to remove fluff.
Result:
Starting
Finally
Error: Finally error (-2700)
NOTE: I usually try to avoid this at all costs, because it hides the real cause of the error. So if I think there's a chance the code in the Finally section could generate an error, I wrap it in a Try/Catch and eat the error:
try {
console.log("Starting");
throw new Error("Example error");
} finally {
try {
console.log("Finally");
throw new Error("Finally error");
} catch {
}
}
console.log("After try/finally");
Try/Catch/Finally Baseline
Nothing unexpected here, other than the "Catch" eats the error.
try {
console.log("Starting");
throw new Error("Example error");
} catch (e) {
console.log("Catch caught this error: " + e.message);
} finally {
console.log("Finally");
}
console.log("After try/finally");
Result:
Starting
Catch caught this error: Example error
Finally
After try/finally
Try/Catch/Finally with Errors
Since the Finally section throws an error, it overrides the errors from the Try and the Catch section.
try {
console.log("Starting");
throw new Error("Example error");
} catch (e) {
console.log("Catch caught this error: " + e.message);
throw e;
} finally {
console.log("Finally");
throw new Error("Finally error");
}
console.log("After try/finally");
Result:
Starting
Catch caught this error: Example error
Finally
Error: Finally error (-2700)
Conclusion:
This is the way Try/Finally has worked in every language I've ever used it in.