The factorial example I just cited above was for illustration only. I don't have such a macro, it was just an example to make a point. But I'll show you the gist of the solution here. And you can ask further questions. Maybe you can even write the factorial macro after my explanation.
The first step is being able to pass the name of the variable as a parameter. I think you know how to do that part. It's just by passing a regular string to a macro.
The second step, inside the macro, is to extract the parameter and put the parameter into a variable. I would typically do it like this for a simple case:
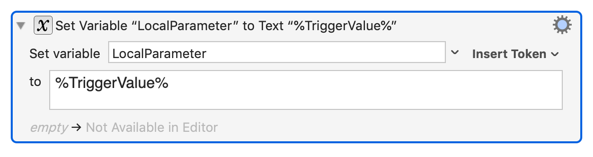
This is one way to get the value of the parameter and put it into a variable. Of course that step can be more complicated if you want to pass multiple data items to the macro. I usually pass two or three variables at a time, but for this example we will pass only one since that's simpler.
Now that you have the contents of the parameter, this step is optional, but you usually want to extract the contents of the variable by that name. Here's how:
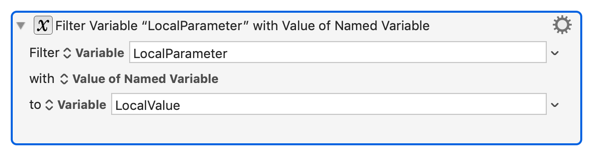
This important step takes the value of the LocalParameter, which in your case might be "Variable1" and puts the contents of the variable "Variable1" into a new local variable called "LocalValue". Back in school we used to call this "dereferencing" a variable. That means taking the reference string "Variable1" and converting it to its contents.
So that's the first part of the battle, getting the contents of the variable inside the macro. Form this point on you use LocalValue as your "copy" of "Variable1". So instead of doing a factorial, let's just square the value because that's simpler:
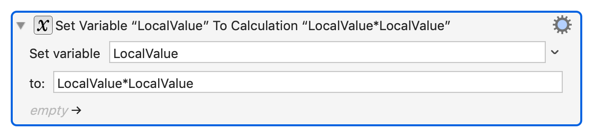
Now for the slightly tricky part: returning the value to the original variable. At the end of your macro, in order to pass the final value of LocalValue back into the REAL variable, we have to use a special action. It looks like this:
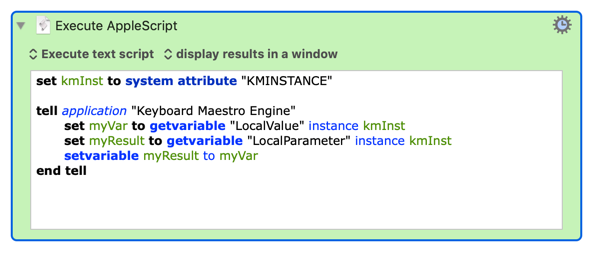
It's worth noting that this action works on KM's local variables only. If your internal variables are global then the syntax will be a little different. I'm recommending that you use local variables for this type of work. But if you insist on using global variable inside your macro we can probably get you the modified syntax.
If you put the above four actions into a macro, let's call it "Square variable", then this is how you would call it:
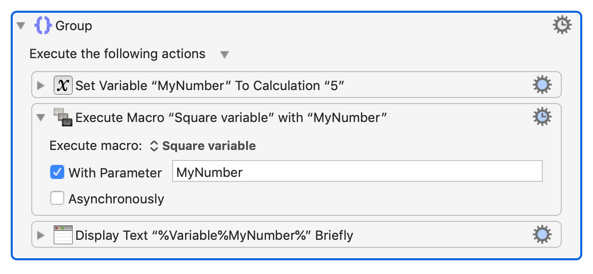
When you run that last block of code outside the macro, it will create a global variable called MyNumber, pass the NAME of the variable to the Squaring macro, (note that it does NOT pass the value, just the name as a string), then when that macro returns the variable MyNumber has miraculously turned into the number 25.
This is a simple example. Sometimes I pass both a variable and a constant to a macro, perhaps like this:
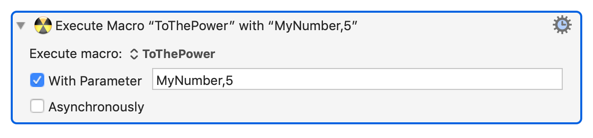
That would require extra handling to separate the variable part of the parameter from the numerical value. Hopefully this gives you the tools you need to try it out for yourself.
By the way, once you master this, we can probably show you how you can pass macro names to macros, not just variable names! I'm working on that because I'm creating a mini-language that KM interprets and my language needs to be able to specify KM macros by name.