I am writing a macro to perform different actions based on the type of text in the clipboard
- If it is all numbers, I want it to open a specific url
- If it is all
text that starts with a http, I want it to open the URL on the
clipboard
- If it is text that doesn’t start with a http, I want it to
open another specific URL.
How do I write this in KBM?
I think what you need is probably 'matches' and a regex or two
Branch on clipboard text pattern.kmmacros (5.3 KB)
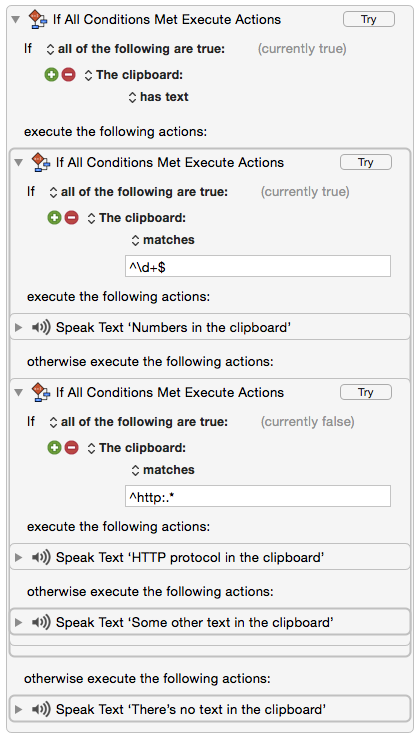
2 Likes
Thanks. Didn’t know one could use regex.
I am thinking that it may be better if the conditions go thus:
If condition 1,
Then …
ELIF condition 2,
Then…
ELSE…
You can nest further IF THEN ELSE structures inside any THEN or ELSE expression (to arbitrary depth) to get any logic that you need.
(The graphic above may look more sequential and less nested than it really is)
Hey Mirizzi,
You can always use AppleScript or the shell to script your IF-ELIF-ELSE structure, and sometimes this is easier to visualize than KM's IF constructs.
Vanilla AppleScript:
----------------------------------------------------------
set clipboardText to the clipboard
if class of clipboardText = text then
tell application "Safari"
activate
if clipboardText starts with "http:" or clipboardText starts with "https:" then
make new document with properties {URL:clipboardText}
else if my allDigits(clipboardText) = true then
set myURL to "http://myDigitUrl.com"
make new document with properties {URL:myURL}
else
set myURL to "http://myTextUrl.com"
make new document with properties {URL:myURL}
end if
end tell
end if
on allDigits(_str)
repeat with i in (characters of _str)
if i is not in {"0", "1", "2", "3", "4", "5", "6", "7", "8", "9"} then
return false
end if
end repeat
return true
end allDigits
----------------------------------------------------------
Applescript REQUIRING the Satimage.osax (link)
------------------------------------------------------------
# REQUIRES the Satimage.osax AppleScript Extension { http://tinyurl.com/dc3soh }
set clipboardText to the clipboard
if class of clipboardText = text then
tell application "Safari"
activate
if my srchBool("^https?:.+", clipboardText, false, true) then # URL
make new document with properties {URL:clipboardText}
else if my srchBool("^\\d+$", clipboardText, false, true) then # NUMBERS
set myURL to "http://myNumbersUrl.com"
make new document with properties {URL:myURL}
else # TEXT
set myURL to "http://myTextUrl.com"
make new document with properties {URL:myURL}
end if
end tell
end if
------------------------------------------------------------
--» HANDLERS
------------------------------------------------------------
on srchBool(regEx, _text)
try
find text regEx in _text with regexp and string result without case sensitive
return true
on error
return false
end try
end srchBool
------------------------------------------------------------
The shell:
clip=$(pbpaste);
if [[ "$clip" =~ ^https?:// ]]; then
open -a Safari "$clip";
elif [[ "$clip" =~ ^[0-9]+$ ]]; then
open -a Safari "http:MyDigitUrl.com";
else
open -a Safari "http:MyTextUrl.com";
fi
-Chris
1 Like
Your knowledge is amazing. Thanks for the code and giving me options. The shell version capped it- so concise, yet fully functional.