var a = { test: "text" };
var b = a;
log(a);
log(b);
function log(obj) {
...
}
What gets passed to “log”? a, b, or { test: “text” }? The answer is { test: “text” }. and { test: “text” } does not have a name property.
Well, I suppose it could have a name property:
var a = { test: "text", name: "ralph" };
… but it wouldn’t have anything to do with the name of the variable. 
By the way, MDN is my go-to reference source.
Assuming you mean a text expansion, I think you're probably right here.
So, I'd enter the object variable name, like "kmResults", and it would type:
logObj(kmResults, "kmResults");
where I have:
function logObj(o, name) {
console.log((name ? name + ": " : "") + JSON.stringify(o, null, 2));
}
Good enough!
I know you guys can, and probably will, write your own macros for this, but for any interested readers, here's my simple macro:
###MACRO: Paste logObject Function in JavaScript
~~~ VER: 1.0 2016-08-25 ~~~
####DOWNLOAD:
Paste logObject Function in JavaScript.kmmacros (3.6 KB)
###ReleaseNotes
TRIGGER: Typed String of:
;lo.ObjectVarName
followed by a [SPACE]
EXAMPLE:
;lo.kmResults
Assumes the log function name is:
logObject(Object, "ObjectName");
###Example Results
logObject(kmResults, "kmResults");
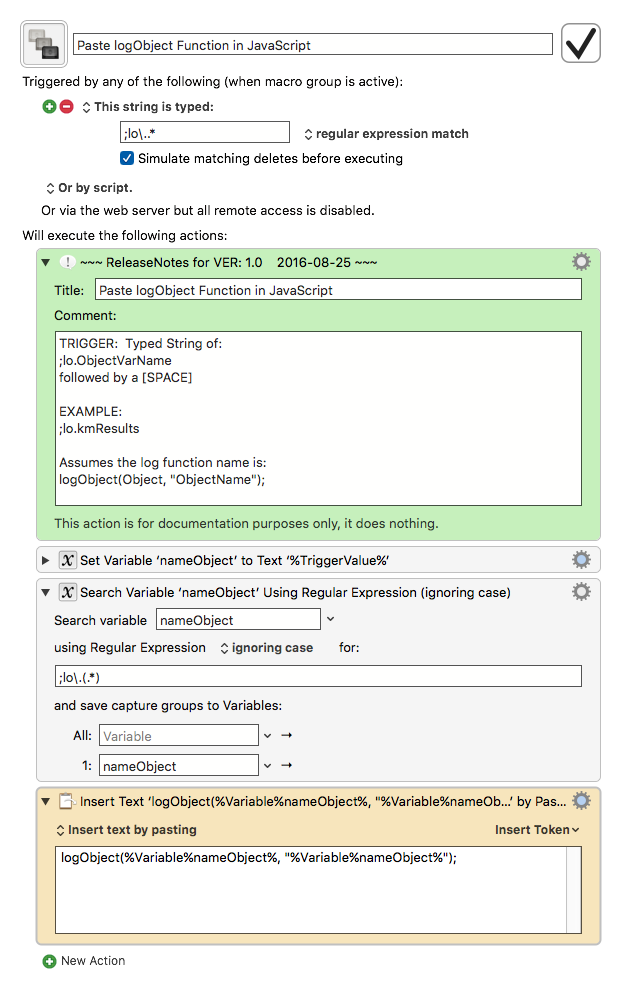
###logObject function
Just for completeness, here's the function I use:
function logObject(pObject, pObjectName) {
console.log(pObjectName + ": " + JSON.stringify(pObject, null, 2));
}