Ah, so "without preceding number/period" was a typo. Gotcha.
Much of KM -- indeed, programming in general -- is taking what you already know/have and tweaking it to fit a particular situation.
There's already a bunch of ways above that will put each line of some text into individual variables. The main difference between the original problem and this is that you only want to process lines that start with one or more numbers then a period.
Two ways of doing that are
-
For each line in the text -- if it starts with numbers and a period then create a new variable and store the line in it. If it doesn't, ignore it
-
For each line in the text that starts with some numbers then a period -- create a new variable and store the line in it
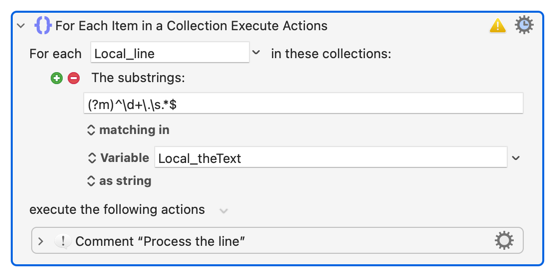
The first builds a Collection containing every line and then works through that Collection looking for matches to process. The second builds a Collection of lines that match and then works through that Collection processing them.
Two approaches to the same problem, both "correct", either can be more "correct" than the other depending on the situation.
Here's the first version in action:
Extract Numeric Lines v3.kmmacros (9.7 KB)
Image
And here's the second:
Extract Numeric Lines v4.kmmacros (8.1 KB)
Image
You can test by changing the number in the final "Display Text" action. And, as before, I've used local variables for testing -- enable the disabled action and disable/delete the action before it to use globals.
There's nothing special about the regex. What is special is the Collection you are creating for the "For Each" action to then work through. Using the above as an example, the action says (ignoring the "item" variable for now):
"Go through the variable Local_theText
and, with ^
and $
matching start- and end-of-lines instead of the start and end of the entire string (the (?m)
, each time you match a substring with some numbers, a period, and a space at the start of the line (^\d+\.\s
) followed by as many any characters (excluding new lines) as possible (.*
) to the end of the line ($
), add that match as a new item to the Collection.
So your Collection starts empty:
{}
...and your regex scans the text from the start, finds the first match, adds a copy of that match to the Collection:
{"1. Introduction"}
(I've used quotes to show that this is an element in the Collection.)
The regex then continues to the next match and adds that:
{"1. Introduction","2. Supplies Needed"}
...and you now have a Collection containing two elements, each a substring of your original text that matches your pattern.
And so on, through the text, until your Collection is
{"1. Introduction","2. Supplies Needed","3. Step-by-Step Cleaning Process","4. Deep Cleaning Tips","5. Additional Maintenance Tips","6. Troubleshooting Common Issues","7. Benefits of Regular Cleaning","8. Conclusion"}
Note that the Collection is "ordered" -- the first item you add is the first item in the Collection, the second the second, etc. It is not sorted, even if the numbers in your example text make it look that way!
All that is mere preparation for the main event -- the magic of "For Each". The action now works through the Collection -- one element at a time, from first to last -- doing whatever is in its "execute" block. So in our example (again, blanking out irrelevant sections):
...the variable Local_line
is set to the first item of the Collection, "1. Introduction" (without the quotes!). The "Set Variable" action evaluates the token in the field for the variable's name -- 01
for the first time through the loop -- and appends that to our literal text "Local_zz_cb" to create the complete name: Local_zz_cb01
, then sets the value of that newly-created variable to the evaluation of the text token %Variable%Local_line%
. We then add 1
to Local_i
.
End result -- we have a variable Local_zz_cb01
with the value "1. Introduction", and Local_i
is 2
.
And we go back to the top of the loop. Local_line
is set to the next item of the Collection, "2. Supplies Needed", and it all happens again but with our new values for Local_line
and Local_i
.
And so on, until all items in the Collection have been processed.
OK -- that's really long-winded (luckily, KM is a lot faster at doing than I am at explaining) but hopefully it makes some sense. If I've confused more than helped then please, please, say -- it'll be a problem with my explaining and not your understanding!